- 06 Feb 2024
- Print
- PDF
Simple Formula Reference
- Updated on 06 Feb 2024
- Print
- PDF
Input and Output
The input value (IV) can use values from the input tag by calling IV or from any other tag by calling IV(tag).
In a PARCview formula, the output value (OV) needs to be specified. This gives more control over the behavior of the tag compared with an Expression. Output is normalized to the timestamps of input tags.
Two useful properties are available to IV and OV:Timestamp and Quality.QualityBits. The .Timestamp property allows retrieving the timestamp of a tag. The .Quality.QualityBits can be used to check the quality of an input value, as IV.Quality.QualityBits. It will return between 0 to 192, A quality of 192 (or higher) is a good quality, anything less than 192 is either uncertain or bad. Some historians such as PI may have different quality values as well, particularly for calculated tags. In order to set OV to a quality other than that of the worst input tag quality, it is necessary to use OV.Quality = Opc.Da.Quality.Good or Opc.Da.Quality.Bad to set the quality as good or bad, respectively.
Variables
Variables can be defined and used in a Formula. These follow VB.NET syntax for dimensioning variables.
Dim Variable As Integer
The most common data types and what they represent are:
Data Type | Declaration |
---|---|
Integer | 8 byte integer |
Long | 16 byte integer |
Single | 8 byte floating point |
Double | 16 byte floating point |
Boolean | True or False |
String | Text |
DateTime | Date and Time |
Variables acting as place holders make code more readable. They also make code easier to debug as it is usually easy to temporarily change the output of a formula to various intermediate variables within a script in an attempt to determine where a calculation flow has gone awry.
Arguments
Parameters can be defined for a script on the Arguments tab. This makes a formula more generalized and therefore significantly more useful as it can be applied to a wider variety of situations.
In order to configure an argument, enter a name and type. The name is what needs to be used a variable in the code, and the type is the data type of the value that gets passed in with the argument.
Arguments can be passed in to a formula in several ways. The values can be entered into the Arguments tab in the Value column.
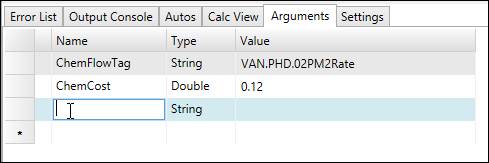
The values can also be entered directly into the formula call in a Trend.

Conditionals and Loops
Conditionals and loops are possible in formulas using VB.NET syntax.
The following block of code shows how to use the If…Then…Else conditional.
' Multiple-line syntax:
If condition Then
[ statements ]
ElseIf elseifcondition Then
[ elseifstatements ]
Else
[ elsestatements ]
End If
' Single-line syntax:
If condition Then [ statements ] [ Else [ elsestatements ] ]
The following block of code shows how to use the For…Next loop.
For i [ As datatype ] = start To end [ Step ]
[ statements ]hi
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ i ]
Filter Formulas
Filters can be applied to tags and formulas using the /FILTER=FilterName() notation. The named formula, or explicit filter, can take zero or more arguments. When building a filter, the Is A Filter box should be checked. This will group it with the filter tags and not formulas when using the filter/formula option in trends.
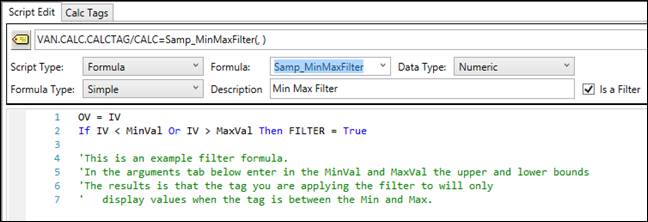
In the following filter, any values of the tag (FilterCriteriaTag) that are less than the MinVal argument or greater than the MaxVal argument are removed from the trend using the FILTER keyword. If FILTER is true for a data point, that point is removed from the trend and the trend interpolates the tag as if that data point does not exist.
OV = IV
If IV < MinVal Or IV > MaxVal Then FILTER = True
An example of this filter applied to the 1-Sine sample tag, with MinVal=3 and MaxVal=4 is shown below on the blue tag.
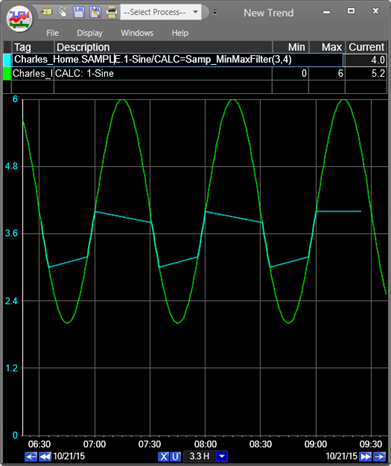
Steam Functions
There are six available functions that can be used to calculate steam properties such as Entropy and Enthalpy using multiple tags.
Available Functions:
TSat(T As Double , TUnits As String , Out As String , OutUnits As String , sPhaseRequest As String )
PSat(P As Double , PUnits As String , Out As String , OutUnits As String , sPhaseRequest As String )
T_P(T As Double , TUnits As String , P As Double , PUnits As String , Out As String , OutUnits As String , sPhaseRequest As String )
P_H(P As Double , PUnits As String , H As Double , HUnits As String , Out As String , OutUnits As String , sPhaseRequest As String )
P_S(P As Double , PUnits As String , S As Double , SUnits As String , Out As String , OutUnits As String , sPhaseRequest As String )
P_X(P As Double , PUnits As String , X As Double , Out As String , OutUnits As String ) As Double
The following output parameters ( Out in the parameters list) are accepted for each function:
TSat | SpecV SatP Enthalpy Entropy |
PSat | SpecV SatT Enthalpy Entropy |
T_P | SpecV Enthalpy Entropy |
P_H | SpecV Temperature Entropy Quality |
P_S | SpecV Temperature Enthalpy Quality |
P_X | SpecV Temperature Enthalpy Entropy |
In the functions the variable P is pressure, T is temperature, X is quality, H is enthalpy, and S is entropy.
The data type of the output of all functions is Double.
The argument sPhaseRequest is looking for V or L (or Vap or Liq), if given one of these it will try to return the vapor or liquid specific property if it makes sense. Leaving it blank will return the two-phase property if the system is two-phase, otherwise the function will return the superheated vapor.
The options for the units are listed below. Every unit is case in-sensitive and space in-sensitive.
Unit Conversion Options
Everything is case in-sensitive and space in-sensitive.
Temperature:
Options | Abbreviation (main) | Alternates |
---|---|---|
Celsius | C | Celsius, DegC, Deg C, Degrees C, Degree C, Centigrade |
Fahrenheit | F | DegF, Deg F, Degrees F, Degree F, Fahrenheit |
Kelvin | K | Kelvin |
Rankine | R | Rankine |
Pressure (Absolute):
Options | Abbreviation (main) | Alternates |
---|---|---|
KiloPascal | kPa | Kilopascal |
MegaPascal | Mpa | Megapascal |
Pounds / Square Inch | PSIA | PSI, lb/in2, #/in2 |
Pounds / Square Foot | PSFA | PSF, lb/ft2, #/ft2 |
Pascal | Pa | Pascal, N/m2 |
Millimeters Mercury | mmHg | mmMercury, Millimeters Mercury |
Inches Mercury | inHg | Inch Hg, Inches Hg, Inches Mercury, Inch Mercury, "Hg,''Hg, In Mercury |
Atmosphere | atm | Atmos, Atmosphere |
Barr | barr | Bar |
Millibarr | mmbarr | mmbar |
Inches Water | InH20 | Inches H20, Inches Water, Inch Water, Inch H20 |
Millimeters Water | mmH20 | mmWater, millimeters H20, millimeters water |
Meters Water | mH20 | mWater, meters H20, meters water |
Torr | torr | |
Millitorr | mtorr | millitorr |
Specific Volume:
Options | Abbreviation (main) | Alternates |
---|---|---|
Meters Cubed / Kilogram | m3/kg | |
Centimeters Cubed / Kilogram | cm3/kg | |
Feet Cubed / Pound Mass | ft3/lbm | ft3/lb |
Liter / Kilogram | L/kg | liter/kg |
Inch Cubed / Pound Mass | in3/lbm | in3/lb |
US Gallon / Pound Mass | gal/lbm | gal/lb |
Specific Enthalpy:
Options | Abbreviation (main) | Alternates |
---|---|---|
Kilojoules / Kilogram | kJ/kg | |
Joules / gram | J/g | |
BTU / Pound Mass | BTU/lbm | BTU/lb |
Kilocalorie / Kilogram | kcal/kg | kcalorie/kg |
calorie / gram | cal/g | calorie/g |
Joules / kilogram | J/kg | |
Therm / Pound Mass | thm/lbm | thm/lb, therm/lbm, therm/lb, therms/lbm, therms/lb |
Therm / Kilogram | thm/kg | therm/kg, therms/kg |
Specific Entropy :
Options | Abbreviation (main) | Alternates |
---|---|---|
Joules / Gram / Kelvin | J/g/K | J/K/g,J/(g K),J/(g*K),J/(K*g) |
BTU / Pound Mass / Rankine | BTU/lbm/R | BTU/lb/R, BTU/R/lbm |
Kilojoules / Kilogram / Kelvin | kJ/kg/K | kJ/K/kg |
Joules / Kilogram / Kelvin | J/kg/K |
Example:
Dim T As Double
Dim P As Double
Dim TUnitsIn As String
Dim PUnitsIn As String
Dim OutValue As String
Dim OutValueUnits As String
Dim PhaseRequest As String
T = IV * 100
P = IV * 300
TUnitsIn = "F"
PUnitsIn = "kPa"
OutValue = "Enthalpy"
OutValueUnits = "BTU/lbm"
PhaseRequest = "" ' If this is V or VAP then Vapor, ir L or LIQ then Liquid, Else return as appropriate
OV = Steam.T_P(T,TUnitsIn,P,PUnitsIn,OutValue,OutValueUnits,PhaseRequest)